Introduction The MAX6950 and MAX6951 are 5-bit and 8-bit common cathode LED display drivers, respectively, controlled by a high-speed SPI interface. These two devices use a unique multiplexing structure, greatly reducing the connection between the LED driver and the LED panel. MAXQ2000 is a high-performance, 16-bit RISC microcontroller with integrated SPI module, which simplifies the connection between the LED driver and the microcontroller. This application note provides a sample MAXQ® assembler to illustrate the use of the MAXQ2000 SPI module with the MAX6951 / MAX6950.
Hardware and software requirements In order to implement the interface test of this application note, a MAX6951 evaluation kit (EV kit), a MAXQ2000 evaluation kit (including MAX-IDE software), a + 5V power supply with a minimum 200mA current, and a PC with an available serial port .
Hardware Settings The MAX6951 EV kit jumper settings remove the jumpers between pins 1 and 2 of JU2, JU3, and JU4, and disconnect the / CS \, DIN, and SCLK signals from the level shifter on the EV kit. The MAXQ2000 EV kit jumper and DIP switch setting switch SW3 pins 1-8 are placed in the off position
JU1: Connect pin 1 and pin 2
JU2: Connect Pin 1 and Pin 2
JU3: Connect Pin 1 and Pin 2
JU4: open circuit
JU10: open circuit
JU11: Turn on (MAXQ2000 evaluation board is powered by a JTAG interface board, the power supply is + 5V.) Connect two evaluation boards as shown in Figure 1.
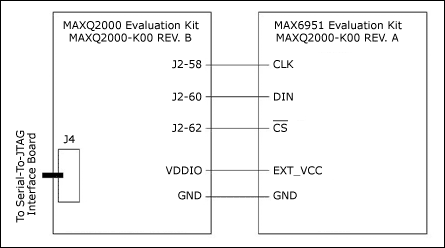
Figure 1. Connection diagram of the MAX6951 evaluation board and the MAXQ2000 evaluation board. Firmware description The complete firmware file for this project can be downloaded from the Maxim website. The integrated development environment and debugging environment provided by Maxim are used for the MAX-IDE of MAXQ series microcontrollers. To compile.
Download: Complete firmware (ZIP, 18.8k)
main.asm file This file is the main loop program of the project. It demonstrates the correct writing of data in the MAX6951 register by calling different subroutines. The firmware demonstrates the following functions of MAX6951: MAX6951 SPI interface initialization. In hexadecimal decoding mode, write and display 0, 1, 2, ..., A, B, C, D, E, F and 8 decimal places (such as , Light up all LED fields). In non-decoding mode, write and display the above letters and other user-built characters, such as H, L, P, Q, Y, etc. LED brightness adjustment. This program explains how to set the MAX6951 brightness register to test the digital brightness control function. Scanning limit cycle program. This program displays the first to eighth digits with the same brightness.
Note: Monitor the process to confirm that when the scanning limit is increased, decrease the brightness. Blink control, the program writes different values ​​to the P0 and P1 levels of each bit of data. You can also use the fast flashing method to demonstrate the segment flashing control function, which can synchronize multiple LED drivers. Scrolling cycle, the program scrolls the text information from left to right and right to left: HELLO. Bounce cycle, the program jumps between the two edges of the LED to display text information: HELLO. Timing cycle, this program explains how to design and display millisecond timing in hexadecimal decoding mode and non-decoding mode. max2000ev_6951.asm file This file contains all the function functions used for communication between the MAX6951 evaluation board and the MAXQ2000 microcontroller. The main functions are: max6951_init: This function sets the SPI mode of MAXQ2000 correctly to achieve the connection with MAX6951. Enable SPI and initialize the MAX6951 to display eight zeros on the display board. Listing 1 is the detailed code of this function.
Listing 1. MAX6951 initialization routine; ****************************************** *************************************; * FuncTIon: max6951_init; *; * Sets the correct SPI modes for talking to the MAX6951, enables SPI, and; *; * iniTIalizes the MAX6951 to display 8 0s.; *; * Input: None.; *; * Output: None.; *; * Destroys: ACC, A [0 ]-A [10], PSF; *; ​​************************************** ***************************************** MAX6951_INIT:; SET SPI BAUD RATE MOVE A [0], # 2400H; SYSTEM CLOCK IS 16,000,000 HZ MOVE A [1], # 00F4H MOVE A [2], # 4240H; DESIRED BAUD RATE IS 1,000,000 HZ MOVE A [3], # 000FH CALL SPI_SETBAUDRATE; SET THE APPROPRIATE MODES FOR THE 6951 MOVE C, #SPI_IDLE_LOW; IDLE = LOW CALL SPI_SETCLOCKPOLARITY MOVE C, #SPI_ACTIVE_EDGE; ACTIVE = RISING EDGE CALL SPI_SETCLOCKPHASE MOVE C, # SPI_LENGTH_16; ALWAYS TRANSFER 16 BITS CALL SPI_SETCHARACTERLENGTH MOVE CASE IS THE SLAVE CALL SPI_SETMODE; ENABLE SPI MOVE C, # 1 CALL SPI_ENABLE ; SHUTDOWN MAX6951 DISPLAY FIRST CALL MAX6951_SHUTDOWN; SET MAX6951 IN HEXADECIMAL DECODE MODE MOVE ACC, # MAX6951REG_DECODE SLA4 SLA4 OR # 0FFH; HEXADECIMAL DECODE CALL MAX6951_TRANSMIT; SET DISPLAY INTENSITY = 16/16 MOVE REC4, # MAX6951 16/16 CALL MAX6951_TRANSMIT; SCAN LIMIT = 7 MOVE ACC, # MAX6951REG_SCANLIMIT SLA4 SLA4 OR # 07H; SCAN LIMIT = 7 CALL MAX6951_TRANSMIT RET max6951_transmit: This function sends a register address and data byte (16 bits) to Max6951 max6951_set_all_n: These functions set the P0 and P1 levels of a digital register to the number "n". All functions have hexadecimal decoding and non-decoding modes. max6951_e_d_s_d: This function first enables the MAX6951 display, then delays by half a second, turns off the display, and then delays by 100ms. max6951_screenshot: These functions display HELLO at eight different positions on the 8-digit LED panel. max6951_scroll_R_to_L: Displayed in different order, this function scrolls HELLO from right to left in a non-decoding manner. max6951_scroll_L_to_R: Display in different order, this function scrolls HELLO from left to right in a non-decoding manner. max6951_bouncing: This function jumps between the two edges of the LED to show HELLO. font_lookup: Given a hexadecimal number, this function queries the same characters displayed on the standard 7-segment LED in a non-decoding manner. max6951_counting: This function is used to display millisecond timing with an accuracy of 10 milliseconds. Listing 2 is the detailed code.
List 2. MAX6951 timing routine; ****************************************** *************************************; * Function: max6951_counting; *; * This routine counts how many 10-milliseconds have elapsed and displays; *; * the value from 0000 to 9999 on LED digits 3-0 (no way to blank leading digits).; *; The routine displays the same value on LED digits 7-4 (by using no decode; *; * mode, individual leading digits can be blanked).; *; * Input: None; *; * Output: None; *; * Destroys: ACC, A [1]-A [4], A [9] ;* ;******************************************** *********************************** MAX6951_COUNTING: CALL MAX6951_SHUTDOWN CALL MAX6951_SET_ALL_0; SET ALL BITS OF DATA REGISTERS TO 0 MOVE ACC, # 010FH; HEXDECIMAL DECODE DIGITS 3-0, NO DEOCDE DIGITS 7-4 CALL MAX6951_TRANSMIT; INITIALIZE THE COUNT TO 0 MOVE A [1], # 0; A [1] => DIGIT 0 MOVE A [2], # 0; A [2] => DIGIT 1 MOVE A [3], # 0; A [3] => DIGIT 2 MOVE A [4], # 0; A [4] => DIGIT 3 COUNT_LOOP: INCREASE_DIGIT3: MOVE ACC, A [4]; PROCESS DIG IT 3 SUB # 9 JUMP Z, INCREASE_DIGIT2; DIGIT 3 = 9, THERE IS CARRY OVER MOVE ACC, A [4]; DIGIT 3 <9, CONTINUE ADD # 1 MOVE A [4], ACC CALL FONT_LOOKUP; LOOK UP THE VALUE FOR THIS FONT; STORE IT IN A [9], KEEP ACC UNCHANGED OR # 6300H CALL MAX6951_TRANSMIT; NO CARRY OVER, WRITE DIGIT 3 NEW VALUE MOVE ACC, A [9]; WRITE THE NO DECODE VALUE TO DIGIT 7 OR # 6700H CALL MAX6951_TRANSMIT JUMP DISPLAY_NUMBER INCREASE_DIGIT2: OR # 6300H CALL MAX6951_TRANSMIT; WRITE 0 TO DIGIT 3 REGISTER FIRST MOVE A [4], # 0; SET DIGIT 3 BACK TO 0 MOVE ACC, # 677EH; NO DECODE VALUE FOR FONT '0' IS "7 "CALL MAX6951_TRANSMIT; WRITE 7EH TO DIGIT 7 REGISTER MOVE ACC, A [3]; PROCESS DIGIT 2 SUB # 9 JUMP Z, INCREASE_DIGIT1; DIGIT 2 = 9, THERE IS CARRY OVER MOVE ACC, A [3]; DIGIT 2 <9 , CONTINUE ADD # 1 MOVE A [3], ACC CALL FONT_LOOKUP; LOOK UP THE VALUE FOR THIS FONT; STORE IT IN A [9], KEEP ACC UNCHANGED OR # 6200H CALL MAX6951_TRANSMIT; NO CARRY OVER, WRITE DIGIT 2 NEW VALUE MOVE ACC, A [9]; WRITE THE NO DECODE VALUE TO DIGIT 6 OR # 6600H CALL MAX6951_TRANSMIT JUMP DISPLAY_NUMBER INCREASE_DIGIT1: OR # 6200H CALL MAX6951_TRANSMIT; WRITE 0 TO DIGIT 2 REGISTER FIRST MOVE A [3], # 0; SET DIGIT 2 BACK TO 0 MOVE ACC, # 667EH; NO DECODE FONT '0' IS "7EH" CALL MAX6951_TRANSMIT; WRITE 7EH TO DIGIT 6 REGISTER MOVE ACC, A [2]; PROCESS DIGIT 1 SUB # 9 JUMP Z, INCREASE_DIGIT0; DIGIT 1 = 9, THERE IS CARRY OVER MOVE ACC, A [ 2]; DIGIT 1 <9, CONTINUE ADD # 1 MOVE A [2], ACC CALL FONT_LOOKUP; LOOK UP THE VALUE FOR THIS FONT; STORE IT IN A [9], KEEP ACC UNCHANGED OR # 6100H CALL MAX6951_TRANSMIT; NO CARRY OVER , WRITE DIGIT 1 NEW VALUE MOVE ACC, A [9]; WRITE THE NO DECODE VALUE TO DIGIT 5 OR # 6500H CALL MAX6951_TRANSMIT JUMP DISPLAY_NUMBER INCREASE_DIGIT0: OR # 6100H CALL MAX6951_TRANSMIT; WRITE 0 TO DIGIT 1 REGISTER FIRST MOVE # 0; SET DIGIT 1 BACK TO 0 MOVE ACC, # 657EH; NO DECODE VALUE FOR FONT '0' IS "7EH" CALL MAX6951_TRANSMIT; WIRTE 7EH TO DIGIT 5 REGISTER MOVE ACC, A [1]; PROCESS DIGIT 0 SUB # 9 JUMP Z, COUNT_COMPLETE; DIGIT 0 = 9, COUNTING IS OVER MOVE ACC, A [1]; DIGIT 0 <9, CONTINUE ADD # 1 MOVE A [1], ACC CALL FONT_LOOKUP; LOOK UP THE VALUE FOR THIS FONT; STORE IT IN A [9], KEEP ACC UNCHANGED OR # 6000H CALL MAX6951_TRANSMIT; NO CARRY OVER, WRITE DIGIT 0 NEW VALUE MOVE ACC, A [9]; WRITE THE NO DECODE VALUE TO DIGIT 4 OR # 6400H CALL MAX6951_TRANSMIT DISPLAY_NUMBER:; DISPLAY DIGIT 3-0 IN HEXADECIMAL DECODE MODE; DIEPLAY DIGIT 7-4 IN NO DECODE MODE CALL MAX6951_ENABLE CALL MAX6951_10MS_DELAY JUMP COUNT_LOOP COUNT_COMPLETE: RET maxq2000_spi.asm file: This file is used to configure and use the MAXQ2000 SPI module. Integrated in MAX-IDE, users can use it without modification.
divide32.asm file: This is a 32-bit division program provided by MAX-IDE software.
maxq2000.inc, maxq2000_spi.inc, and max2000ev_6951.inc files: These are nested files for MAXQ2000 pin definitions and MAX6951 register definitions.
Conclusion The MAX6951 / MAX6950 SPI LED driver is a simple and easy-to-use common cathode display driver that is connected to a microcontroller via an SPI serial interface. The MAXQ series microcontrollers integrate an SPI module, which can communicate with the LED driver through the SPI interface. The routine introduced here helps users to understand the MAX6951 LED drive function. The routine can also be applied to the development of similar MAXQ2000 systems.
Electronic Drawing tablet Features: (drawing with pressure, slim and convenience, erase button, environment-friendly, sturdy and durable)
1.Non-toxic and environment-friendly health eraser board: it can be used as an educational toy, dictation board, calculation paper, drawing board, message board
2. No oil, no ink, dust, pressure painting, the key to clear, environment-friendly health, anti-fall-resistance
3. Reuse, save resources, save 20 trees of 10 age trees in one lifetime, add green for the earth
Digital drawing tablet Instructions:
1. Using the pen's own pen to write and draw, different pen pressures produce different line thicknesses2. Press the clear key to clear the contents of the tablet
When cleared, the LCD Drawing Pad Tablet screen will flicker
12 Inches LCD Writing Tablet,LCD Tablet,LCD Writing Board,Digital Handwriting Pads
Shenzhen New Wonderful Technology Co., Ltd. , https://www.sznewwonderful.com